A directed graph, also known as a digraph, is a collection of vertices (or nodes) connected by edges that have a direction. In Python, you can use the NetworkX library to create and manipulate directed graphs. To create a directed graph, you can use the following code:
python import networkx as nx # Create a directed graph G = nx.DiGraph()
You can then add vertices to the graph using the `add_node()` method and edges using the `add_edge()` method:
python # Add vertices to the graph G.add_node(‘A’) G.add_node(‘B’) # Add edges to the graph G.add_edge(‘A’, ‘B’) G.add_edge(‘B’, ‘C’)
Directed graphs are useful for representing relationships between objects that have a direction. For example, you could use a directed graph to represent the relationships between web pages, where each page is a vertex and the links between pages are edges.
Here are some of the benefits of using directed graphs:
- They can be used to represent complex relationships between objects.
- They can be used to model systems that change over time.
- They can be used to find the shortest path between two vertices.
how to make directed graph in python leetcode
Directed graphs are a powerful data structure that can be used to represent a wide variety of relationships between objects. They are particularly useful for representing networks, such as social networks, transportation networks, and computer networks. In this article, we will discuss the essential aspects of directed graphs and how to create and use them in Python.
- Definition: A directed graph is a collection of vertices (or nodes) connected by edges that have a direction.
- Creation: In Python, you can use the NetworkX library to create and manipulate directed graphs.
- Vertices: Vertices are the individual objects that make up a graph.
- Edges: Edges are the connections between vertices.
- Direction: Edges in a directed graph have a direction, meaning that they can only be traversed in one direction.
- Applications: Directed graphs are used in a wide variety of applications, such as social network analysis, transportation planning, and computer networking.
Here is a simple example of a directed graph that represents a social network:
A -> B B -> C C -> A
In this graph, the vertices represent people and the edges represent friendships. The arrow on each edge indicates the direction of the friendship. For example, the edge from A to B indicates that A is friends with B.
Definition
This definition is essential for understanding how to make a directed graph in Python using the NetworkX library. A directed graph is a data structure that consists of a set of vertices and a set of edges that connect the vertices. Each edge has a direction, meaning that it can only be traversed in one direction. In NetworkX, you can create a directed graph using the DiGraph() function. Once you have created a directed graph, you can add vertices and edges to it using the add_node() and add_edge() methods, respectively.
Directed graphs are useful for representing a wide variety of relationships between objects. For example, you could use a directed graph to represent the relationships between web pages, where each page is a vertex and the links between pages are edges. You could also use a directed graph to represent the relationships between people in a social network, where each person is a vertex and the friendships between people are edges.
Understanding the definition of a directed graph is essential for being able to create and use directed graphs in Python. Directed graphs are a powerful data structure that can be used to represent a wide variety of relationships between objects.
Creation
The NetworkX library is a powerful tool for creating and manipulating graphs in Python. It provides a wide range of functions for creating different types of graphs, including directed graphs. To create a directed graph using NetworkX, you can use the DiGraph() function. This function takes a list of nodes as an argument and creates a directed graph with those nodes. Once you have created a directed graph, you can add edges to it using the add_edge() function. This function takes two nodes as arguments and adds an edge between them.
The ability to create and manipulate directed graphs is essential for solving a wide range of problems in computer science. For example, directed graphs can be used to represent social networks, transportation networks, and computer networks. By understanding how to create and manipulate directed graphs, you can develop powerful algorithms for solving problems in these domains.
Here is an example of how you can use NetworkX to create and manipulate a directed graph:
import networkx as nx # Create a directed graph G = nx.DiGraph() # Add nodes to the graph G.add_nodes_from(['A', 'B', 'C']) # Add edges to the graph G.add_edges_from([('A', 'B'), ('B', 'C'), ('C', 'A')]) # Print the graph print(G.edges())
This code creates a directed graph with three nodes and three edges. The edges are printed to the console, showing the direction of each edge.
Understanding how to create and manipulate directed graphs is an essential skill for any computer scientist. By mastering this skill, you will be able to develop powerful algorithms for solving a wide range of problems.
Vertices
In the context of “how to make directed graph in python leetcode”, vertices play a crucial role as the fundamental building blocks of a directed graph. A directed graph is a collection of vertices connected by edges that have a direction. Therefore, understanding the concept of vertices is essential for effectively creating and manipulating directed graphs in Python.
-
Components of Vertices:
Vertices in a directed graph represent individual objects or entities. They can be of any type, such as strings, integers, or even complex data structures. Vertices serve as the endpoints of edges, providing a foundation for establishing connections and relationships within the graph.
-
Creating Vertices:
In Python using the NetworkX library, vertices can be created and added to a directed graph using the add_node() method. This method takes a single argument, which is the vertex to be added. Vertices can be added one at a time or in bulk using the add_nodes_from() method.
-
Vertex Attributes:
Vertices in a directed graph can have attributes associated with them. These attributes can be used to store additional information or properties about the vertex. In NetworkX, vertex attributes can be set using the set_node_attributes() method.
-
Vertex Connectivity:
The connections between vertices in a directed graph are established through edges. Edges specify the direction and relationship between vertices. Understanding vertex connectivity is crucial for analyzing the flow and relationships within the graph.
In summary, vertices are the fundamental components of a directed graph, representing the individual objects or entities involved. They provide the foundation for establishing connections and relationships through edges, enabling the representation of complex networks and systems in Python using the NetworkX library.
Edges
In the context of “how to make directed graph in python leetcode”, edges play a crucial role in establishing connections and relationships between vertices. A directed graph consists of a set of vertices connected by directed edges, where each edge has a direction and points from one vertex to another. Understanding the concept of edges is essential for effectively creating and manipulating directed graphs in Python using the NetworkX library.
-
Components of Edges:
Edges in a directed graph represent the connections between vertices. They consist of a start vertex and an end vertex, indicating the direction of the relationship. Edges can be weighted or unweighted, with weights representing the strength or cost of the connection. -
Creating Edges:
In Python using NetworkX, edges can be created and added to a directed graph using the add_edge() method. This method takes two arguments, which are the start vertex and the end vertex of the edge. Edges can be added one at a time or in bulk using the add_edges_from() method. -
Edge Attributes:
Edges in a directed graph can have attributes associated with them. These attributes can be used to store additional information or properties about the edge, such as its weight or capacity. In NetworkX, edge attributes can be set using the set_edge_attributes() method. -
Edge Connectivity:
Edges determine the connectivity and flow within a directed graph. They establish the relationships and dependencies between vertices, enabling the analysis of paths, cycles, and other graph properties. Understanding edge connectivity is crucial for exploring the structure and dynamics of the graph.
In summary, edges are the fundamental connections between vertices in a directed graph, providing the means to establish relationships and represent complex networks and systems. By understanding the components, creation, attributes, and connectivity of edges, you can effectively create and manipulate directed graphs in Python using the NetworkX library.
Direction
In the context of “how to make directed graph in python leetcode”, understanding the concept of directionality is crucial for effectively creating and manipulating directed graphs. A directed graph is a collection of vertices connected by edges that have a specified direction. This directionality adds an extra layer of complexity compared to undirected graphs, where edges do not have a defined direction.
The directionality of edges in a directed graph introduces the concept of predecessors and successors. For a directed edge from vertex A to vertex B, vertex A is the predecessor of vertex B, and vertex B is the successor of vertex A. This directional relationship allows for the representation of real-world scenarios where the order of traversal matters.
One practical application of directed graphs is in modeling transportation networks, where roads and streets can be represented as directed edges. By considering the directionality of edges, it becomes possible to analyze traffic flow, identify potential bottlenecks, and plan for efficient routing. Another example is in social networks, where directed edges can represent the follow relationships between users. This information can be used to study the spread of information, identify influential users, and make recommendations.
To create a directed graph in Python using the NetworkX library, the DiGraph() function is used. This function takes a list of nodes as input and creates a directed graph with those nodes. Once the graph is created, edges can be added using the add_edge() method, which takes two nodes as arguments and adds a directed edge from the first node to the second node.
In summary, understanding the concept of directionality in edges is essential for effectively creating and manipulating directed graphs in Python. Directed graphs are powerful data structures that can be used to model a wide range of real-world scenarios where the order of traversal matters.
Applications
Directed graphs are a powerful tool for representing and analyzing relationships between objects. They are used in a wide variety of applications, including:
- Social network analysis: Directed graphs can be used to represent the relationships between people in a social network. The nodes in the graph represent people, and the edges represent the relationships between them. Directed graphs can be used to analyze the structure of social networks, identify influential individuals, and study the spread of information.
- Transportation planning: Directed graphs can be used to represent transportation networks, such as road networks and public transportation systems. The nodes in the graph represent locations, and the edges represent the roads or public transportation routes that connect them. Directed graphs can be used to analyze the efficiency of transportation networks, identify bottlenecks, and plan for future improvements.
- Computer networking: Directed graphs can be used to represent computer networks, such as the Internet. The nodes in the graph represent computers or other devices, and the edges represent the network connections between them. Directed graphs can be used to analyze the performance of computer networks, identify potential problems, and plan for future upgrades.
These are just a few examples of the many applications of directed graphs. By understanding how to make directed graphs in Python, you can use them to solve a wide variety of problems in different domains.
FAQs on How to Make Directed Graphs in Python
This section provides answers to some frequently asked questions about directed graphs in Python, offering insights and clarifying common misconceptions.
Question 1: What is the difference between a directed and an undirected graph?
A directed graph differs from an undirected graph in that its edges have a specified direction. In a directed graph, edges indicate a one-way relationship between vertices, whereas in an undirected graph, edges represent a bidirectional relationship.
Question 2: How do I create a directed graph in Python?
To create a directed graph in Python using the NetworkX library, you can use the `DiGraph()` function. This function takes a list of nodes as input and creates a directed graph with those nodes. You can then add edges to the graph using the `add_edge()` method.
Question 3: How do I add edges to a directed graph?
To add edges to a directed graph in Python using NetworkX, you can use the `add_edge()` method. This method takes two arguments: the source node and the target node. The source node is the node from which the edge originates, and the target node is the node to which the edge points.
Question 4: How do I find the shortest path between two nodes in a directed graph?
To find the shortest path between two nodes in a directed graph in Python using NetworkX, you can use the `shortest_path()` function. This function takes two arguments: the source node and the target node. It returns a list of nodes that represents the shortest path from the source node to the target node.
Question 5: What are some applications of directed graphs?
Directed graphs have a wide range of applications, including social network analysis, transportation planning, and computer networking. In social network analysis, directed graphs can represent the relationships between people, where the edges indicate the direction of the relationship (e.g., friendship, following). In transportation planning, directed graphs can represent road networks, where the edges indicate the direction of traffic flow. In computer networking, directed graphs can represent network topologies, where the edges indicate the direction of data flow.
Question 6: What are some resources for learning more about directed graphs in Python?
There are several resources available for learning more about directed graphs in Python. The NetworkX documentation provides a comprehensive overview of the library’s functionality, including its support for directed graphs. Additionally, there are numerous tutorials and online courses that cover the basics of directed graphs and how to use NetworkX to manipulate them.
Remember, understanding directed graphs and their applications is essential for tackling various problems in computer science and data analysis. By leveraging the power of directed graphs and the NetworkX library, you can effectively model and analyze complex systems and relationships.
For further exploration, refer to the provided resources mentioned in the last FAQ.
Tips on Making Directed Graphs in Python
Directed graphs are a powerful tool for representing and analyzing relationships between objects. They are used in a wide variety of applications, including social network analysis, transportation planning, and computer networking. If you are working with directed graphs in Python, here are a few tips to help you get started:
Tip 1: Use the NetworkX library.
NetworkX is a powerful Python library for working with graphs. It provides a wide range of functions for creating, manipulating, and analyzing graphs, including directed graphs. If you are new to working with directed graphs in Python, I recommend starting with NetworkX.
Tip 2: Understand the difference between directed and undirected graphs.
Directed graphs differ from undirected graphs in that their edges have a specified direction. In a directed graph, edges indicate a one-way relationship between vertices, whereas in an undirected graph, edges represent a bidirectional relationship. It is important to understand this difference before you start working with directed graphs.
Tip 3: Use the DiGraph() function to create a directed graph.
To create a directed graph in Python using NetworkX, you can use the DiGraph() function. This function takes a list of nodes as input and creates a directed graph with those nodes. You can then add edges to the graph using the add_edge() method.
Tip 4: Use the add_edge() method to add edges to a directed graph.
To add edges to a directed graph in Python using NetworkX, you can use the add_edge() method. This method takes two arguments: the source node and the target node. The source node is the node from which the edge originates, and the target node is the node to which the edge points.
Tip 5: Use the shortest_path() function to find the shortest path between two nodes in a directed graph.
To find the shortest path between two nodes in a directed graph in Python using NetworkX, you can use the shortest_path() function. This function takes two arguments: the source node and the target node. It returns a list of nodes that represents the shortest path from the source node to the target node.
Summary:
Directed graphs are a powerful tool for representing and analyzing relationships between objects. By understanding the tips outlined above, you can effectively create, manipulate, and analyze directed graphs in Python using the NetworkX library.
Conclusion
In this article, we have explored the topic of “how to make directed graph in python leetcode”. We have discussed the definition of a directed graph, how to create and manipulate directed graphs using the NetworkX library in Python, and the various applications of directed graphs. We have also provided some tips on how to effectively work with directed graphs in Python.
Directed graphs are a powerful tool for representing and analyzing relationships between objects. They are used in a wide variety of applications, including social network analysis, transportation planning, and computer networking. By understanding how to make directed graphs in Python, you can use them to solve a wide range of problems in different domains.
Youtube Video:
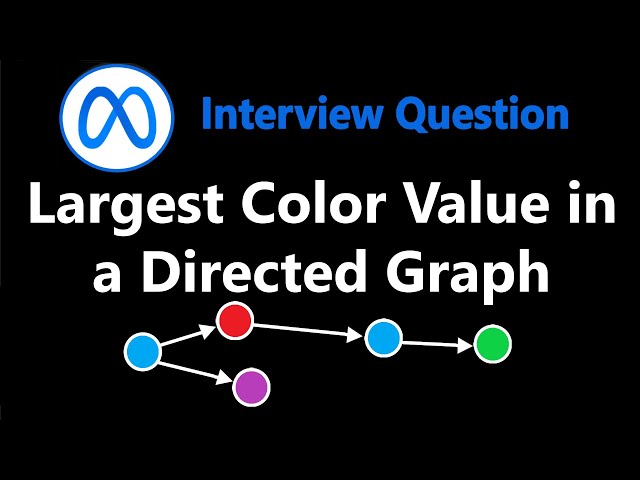